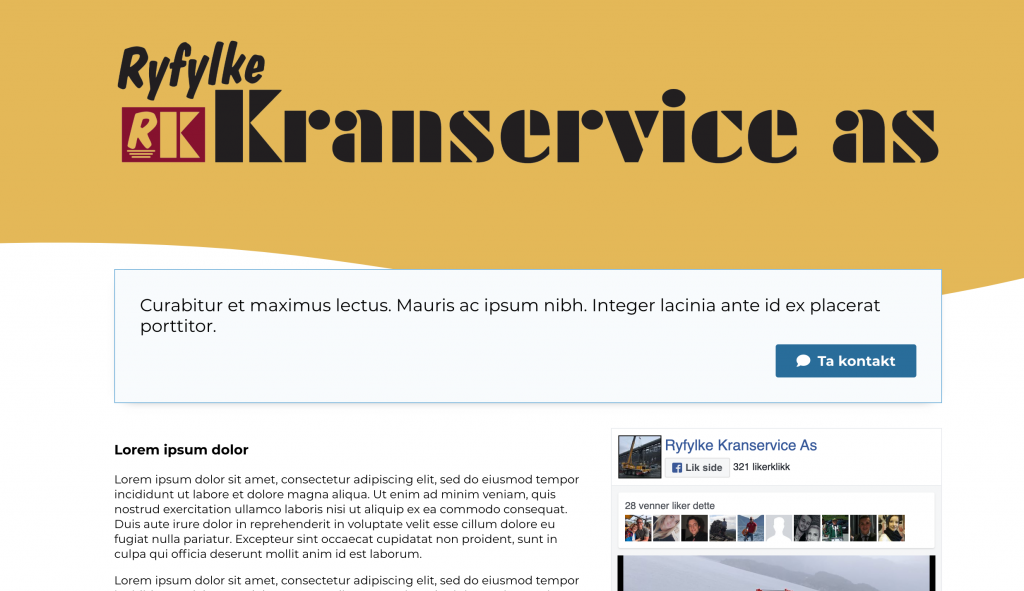
My latest project has been developing Ryfylke Kranservice as’s website. They wanted a simple website, with a simple back-system for them to edit the website. I decided to go for React again for this project, mainly for the benefits of maintenance, but also the performance.
Drag ‘n’ drop editing
This was my first introduction to building a drag ‘n’ drop feature in React. The website includes a gallery, and to allow the owner to easily reorder the gallery I decided on creating a drag ‘n’ drop feature. To my surprise it was pretty easy and went very well.
I use the following props/event-listeners on the image objects:
- onDrag: When item is dragged
- onDrop: When something is dropped on top of the item.
- onDragOver: When something is dragged over the item.
- onDragLeave: When something that is dragged over the item leaves the item.
- data-priority: Numeric order of objects
- key: index
Here are my event functions:
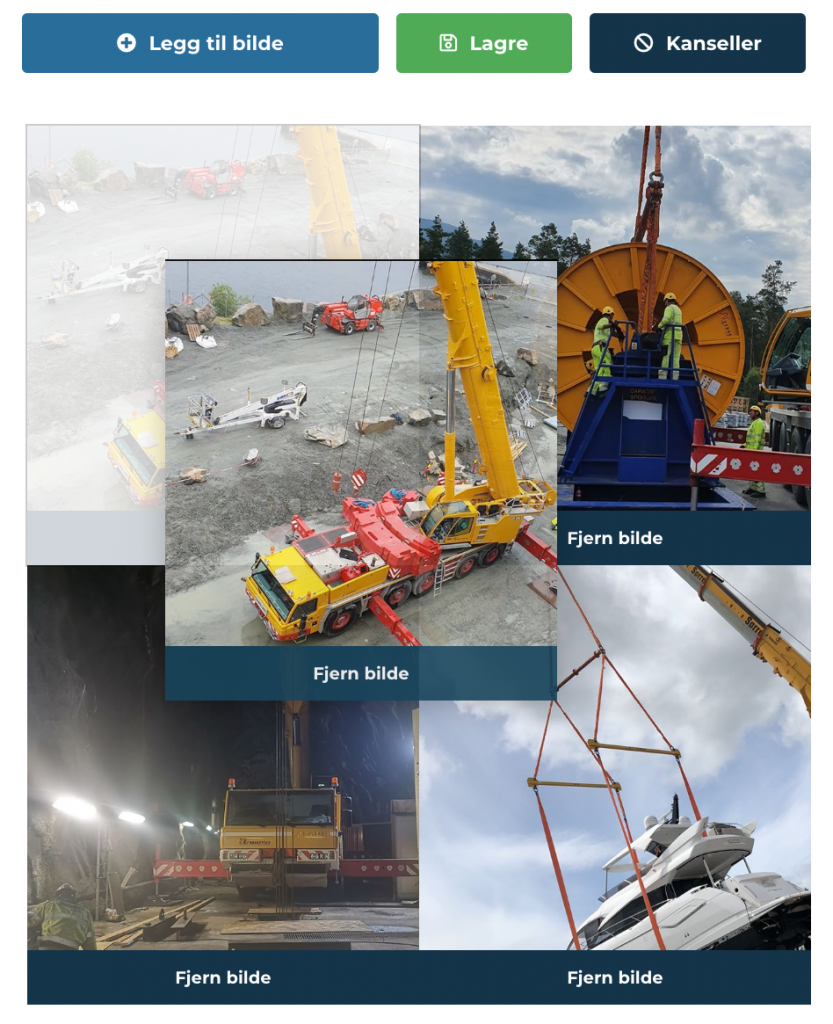
onDrag = event => {
event.preventDefault();
this.setState({
draggedItem: event.target.getAttribute("data-priority")
});
};
onDragOver = event => {
event.preventDefault();
event.target.classList.add("draggedOver");
};
onDragLeave = event => {
event.preventDefault();
event.target.classList.remove("draggedOver");
};
onDrop = event => {
event.preventDefault();
let droppedOnto = parseInt(event.target.getAttribute("data-priority"));
let droppedItem = parseInt(this.state.draggedItem);
let newImages = [];
this.state.images.forEach(img => {
let url = img.url;
let priority = img.priority;
// Here is where I swap places on drop //
if (priority === droppedItem) {
priority = droppedOnto;
} else if (priority === droppedOnto) {
priority = droppedItem;
}
/////////////////////////////////////////
newImages.push({ url, priority });
});
this.setState({
images: sortObjects(newImages)
});
event.target.classList.remove("draggedOver");
};