Hi! It’s been a while. I wanted to write an article to explain what I’ve been up to lately, and share some hopefully interesting thoughts.
Yearly write up
Hopefully, I’ll be able to write more than one article next year. It’s been busy for sure, and just really a big change of scene. When covid first arrived, I had just started working at Idean. Now – almost two years later(!), a lot of things have changed.
Starting my own company
So I started a company, Ryfylke React AS. It came about mostly as a means to an end when I got a possible offer to work on a new project over at Telenor. The offer went through and I’ve now been working at said project on behalf of my own company for almost a year (since February 2021).
Starting the company has been an interesting process. There are obviously lots of logistics to take care of, taxes, stuff like that – but it’s mostly gone smooth. The independence and economic stability that I’ve gained has far outweighed the cost of the time I’ve spent doing work unrelated to web development.
The project
One of the reasons I’ve been so silent over here for the past year has been the strict NDA policy. I won’t be able to share any of the specifics, but I can delve into some interesting challenges that I’ve met – and so I figured I’d use this article to do exactly that.
🤫
Scaling up
This project is definitely the most complex and large-scale project I’ve worked on so far. It involves a lot of graph work, data visualisation and interesting components. One thing I’ve really enjoyed, is having more of a say in how the code is set up and organised. We have a great setup for working with styling, forms, the API and testing – all very important things on large-scale projects. On that note…
Javascript testing
This was honestly a subject I wasn’t very experienced at last year – but something I now feel a lot more comfortable with. I wouldn’t say that we necessarily use test-driven development, yet, in our case it was more of a necessity that we’ve been working at after the fact.
We started out with functional tests, using Testcafè. I’ve enjoyed the developer experience working with this library, and it’s worked well for our setup. Most of our pages have a dedicated file with functional tests related to it. Lately, we’ve also started writing unit tests for our components, using Jest and React Testing Library. The flow and syntax is very similar to Testcafè, which has made that transition pretty smooth and painless so far.
Further developing my “stack”
As a developer (and human), your opinions are constantly flavoured by your experience. These are some of the technologies that I’ve found really great, and that I don’t see myself swapping out voluntarily in the near future.
🔥 react-hook-form
I can’t say enough good about this library. It’s a blast to work with, it’s easy to implement into any component library and it does a really good job of keeping your codebase clean, yet still giving you powerful utils. Coupled with yup for validation, react-hook-form has truly made coding forms a much better experience for me.
🔥 Redux toolkit
Has made working with redux (and especially async and API logic in conjunction with redux) a breeze. Love it.
🔥 styled-components
I have mostly thrown away classnames for styling in favour of writing styled components. Makes the markup real pretty and lets me essentially write SCSS, but with some powerful Javascript coupling through props.
And more…
I’ll make a separate post about my full stack that can evolve separately from this article, I just wanted to share some thoughts I’ve picked up over the last year.
Open source work
I’ve finally popped my cherry on open source work as well, mostly on Carbon Design System. I’ve opened a few issues that I encountered when using their components – participated in discussions, and even made a pull request related to one of my issues.
The team over at IBM has been very helpful and welcoming – and I’ve enjoyed the experience. I’ve always been a big fan of open source as a concept and I’m glad I’ve finally had the chance to start making a meaningful contribution. This is something I hope to do more of in 2022.
What else…
Well, I moved back home to Suldal, in with my girlfriend. That’s a pretty big one. Lovely place, I’ve gotta say. We have two cats. Molly I’ve had for several years now, but Luci is just barely a year old – and he’s technically my girlfriend’s cat. They get along alright, better now than in the beginning – had to have them both neutered.
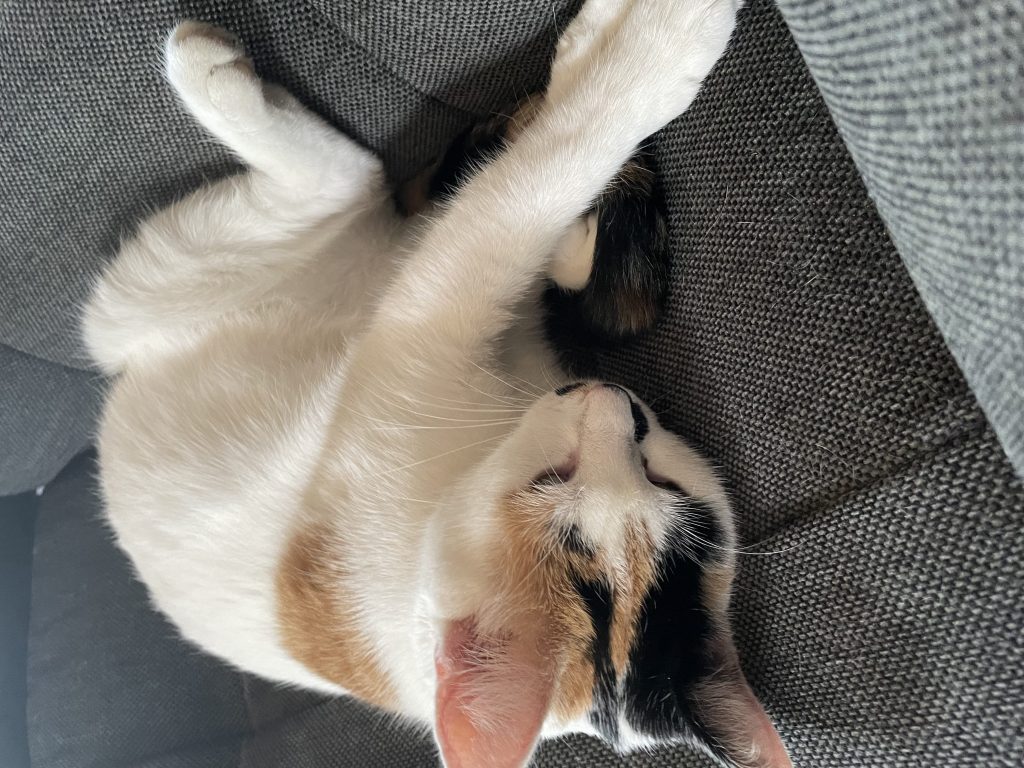
I guess to summarise, I’ve had a really busy but really amazing year. I’ve learned lots of interesting stuff and developed as a developer – and I’ve also made big changes in my personal life. All in all, I’m happy with how things have gone and I’m looking forward to next year.
Hopefully not until then,
Håkon.